WebSockets for Beginners: A Real-Time Revolution with Socket.IO
The standard web operates on a request-response model.
This means your web browser (the client) sends a request to a server, and the server responds. This works for many scenarios, but what about applications where you need the server to push updates to the client without the client explicitly asking for them? Think chat applications, stock tickers, or multiplayer games. This is where WebSockets shine!
What are WebSockets?
- Persistent Connection: WebSockets establish a full-duplex, persistent connection between a client and a server. Once open, this connection allows both parties to send data back and forth at any time.
- Real-time Power: This eliminates the need for clients to constantly poll the server for updates, leading to incredibly low-latency, real-time communication.
- Beyond HTTP: WebSockets use their own protocol (starting with an HTTP handshake), enabling features not possible with traditional HTTP.
Socket.IO to the Rescue
Socket.IO is a powerful JavaScript library that simplifies WebSocket implementation on both the frontend (client-side) and backend (server-side). It provides:
- Abstraction: Handles the complexities of WebSockets, giving you a clean event-based interface.
- Reliability: Offers fallbacks for browsers without WebSocket support.
- Features: Includes features like rooms (for grouping clients) and broadcasting (sending messages to multiple clients).
Getting Started
-
Project Setup (Node.js Backend)
- Create a Node.JS project (
npm init -y
) - Install Socket.IO:
npm install socket.io
- Create a Node.JS project (
-
Basic Server
JavaScriptconst express = require('express'); const app = express(); const http = require('http').createServer(app); const io = require('socket.io')(http); io.on('connection', (socket) => { console.log('A user connected'); socket.on('disconnect', () => { console.log('A user disconnected'); }); }); http.listen(3000, () => { console.log('Server listening on port 3000'); });
-
Simple Client (HTML)
-
Core Concepts: Events and Communication
-
Emitting Events: Sending messages with a specific name.
- Client:
socket.emit('chat message', 'Hello from the client!');
- Server:
io.emit('new message', 'Hello from the server!');
- Client:
-
Listening to Events: Reacting to received messages.
- Client:
socket.on('new message', (data) => { console.log(data); });
- Server:
socket.on('chat message', (data) => { console.log(data); });
- Client:
Building a Real-Time Chat App (Example)
Enhance the provided HTML and JavaScript to implement a basic chat application where users can send and receive messages.
Resources
- Socket.IO Docs: https://socket.io/get-started/chat
- WebSockets on MDN: https://developer.mozilla.org/en-US/docs/Web/API/WebSockets_API
- YouTube Tutorials: Search for "WebSocket Socket.IO tutorials"
Let the Real-Time Fun Begin!
This is just the beginning! Explore broadcasting messages to rooms, user authentication, and more. WebSockets and Socket.IO open a world of possibilities for building dynamic, real-time web experiences.
What's Your Reaction?
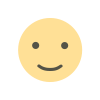
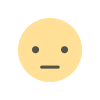
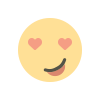
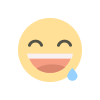
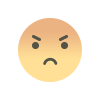
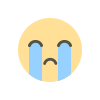
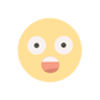