Unleashing the Power of Laravel: A Comprehensive Guide to Its Benefits and Real-World Examples
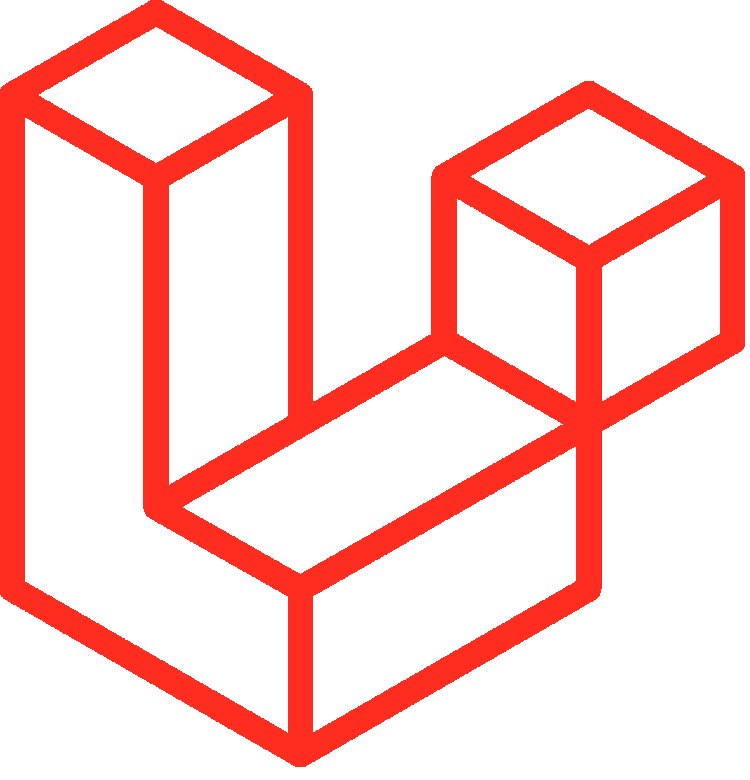
In the vast landscape of web development, choosing the right framework can be a daunting task. With an array of options available, it's essential to select a framework that not only streamlines your development process but also provides a robust and efficient solution. Laravel, a free, open-source PHP web application framework, has emerged as a popular choice for developers worldwide. In this extensive guide, we'll delve deep into the benefits of using Laravel, backed by real-world examples that highlight its capabilities.
Table of Contents
-
A Brief Introduction to Laravel
- What is Laravel?
- History and Evolution
-
Why Choose Laravel?
- The Laravel Ecosystem
- Modern PHP Development
- Robust Security Measures
-
Key Benefits of Using Laravel
a. Elegant Syntax and Expressive Code b. Modular Architecture and Packages c. Built-in Tools and Features d. Blade Templating Engine e. Eloquent ORM f. Migrations and Database Management g. Artisan Console h. RESTful Routing and Controllers i. Authentication and Authorization j. Testing and Debugging
-
Real-World Examples of Laravel Applications
a. E-commerce Platforms b. Content Management Systems (CMS) c. Social Media Platforms d. Enterprise Resource Planning (ERP) Systems e. Educational Portals f. Project Management Tools g. Healthcare Information Systems h. Travel and Booking Applications i. Startups and MVPs j. Ecosystem and Community Projects
-
Performance Optimization in Laravel
- Caching and Performance Enhancement
- Database Query Optimization
- Scalability and Load Balancing
-
Security Features and Best Practices
a. Authentication and Authorization b. Input Validation c. Cross-Site Request Forgery (CSRF) Protection d. Cross-Site Scripting (XSS) Prevention e. SQL Injection Prevention f. Rate Limiting and Throttling g. Security Updates and Patches
-
Integrating Laravel with Other Technologies
a. Laravel and JavaScript Frameworks (Vue.js, React) b. RESTful APIs and Microservices c. Docker and Containerization d. Cloud Services (AWS, Azure, GCP) e. CI/CD Pipelines
-
Extending Laravel with Composer Packages
a. Awe-Inspiring Laravel Packages b. Installation and Usage
-
Laravel's Role in IoT and Real-Time Applications
a. WebSockets and Broadcasting b. IoT Data Collection and Management c. Real-Time Notifications
-
Continuous Learning and Community Support
a. Laravel Documentation b. Online Courses and Tutorials c. Laravel Conferences and Events d. Active Forums and Community Resources
- Case Studies: Success Stories with Laravel
a. Taylor Otwell's Journey b. Laravel-Powered Startups c. Large-Scale Applications
- The Future of Laravel
a. Upcoming Features b. Trends in Web Development
- Conclusion
a. Recap of Benefits and Real-World Examples b. The Ongoing Relevance of Laravel c. Your Next Steps
Chapter 1: A Brief Introduction to Laravel
What is Laravel?
Laravel, created by Taylor Otwell, is a PHP web application framework designed to make web development easier, more efficient, and enjoyable. It follows the Model-View-Controller (MVC) architectural pattern, providing a structured foundation for building web applications.
Laravel's primary goal is to simplify common tasks such as routing, caching, authentication, and database management, allowing developers to focus on writing clean and expressive code. The framework is open-source and built on top of various Symfony components, making it both powerful and extensible.
History and Evolution
Laravel was first released in 2011, and since then, it has gone through multiple versions and continuous improvement. The evolution of Laravel includes:
- Laravel 1.0 (June 2011): The initial release was a basic framework with minimal features.
- Laravel 2.0 (September 2011): This version introduced support for the Blade templating engine.
- Laravel 3.0 (February 2012): Laravel 3 marked a significant change in the framework's architecture, bringing in bundles and more flexibility.
- Laravel 4.0 (May 2013): This version emphasized Composer integration, making package management seamless.
- Laravel 5.0 (February 2015): Laravel 5 introduced the Laravel Elixir asset management tool and improved support for asynchronous programming.
- Laravel 6.0 (September 2019): Laravel 6 came with Laravel Vapor, a serverless deployment platform for Laravel applications.
- Laravel 7.0 (March 2020): Laravel 7 introduced better support for custom Eloquent cast types and Laravel Airlock for API authentication.
- Laravel 8.0 (September 2020): This version included the introduction of model factories and improved job batching.
- Laravel 9.0 (TBD): While the exact features of Laravel 9 are yet to be confirmed, it is expected to continue the tradition of innovation and improvement.
Laravel has gained immense popularity over the years, thanks to its modern development practices, robust security measures, and a thriving community of developers. In the following chapters, we will explore in detail why Laravel has become the go-to choice for web developers across the globe.
Chapter 2: Why Choose Laravel?
The Laravel Ecosystem
Laravel is not just a framework; it's an entire ecosystem that includes a wide range of tools, packages, and resources to support web development. The Laravel ecosystem comprises:
- Laravel Nova: A powerful administration panel for managing resources, models, and data.
- Laravel Forge: A server management tool that simplifies server provisioning, deployment, and scaling.
- Laravel Vapor: A serverless deployment platform for Laravel applications, designed to handle auto-scaling and infrastructure management.
- Laravel Envoyer: A tool for zero-downtime deployment and advanced deployment features.
- Laravel Spark: A scaffolding tool for creating SaaS applications.
- Laravel Mix: An asset compilation and build tool for CSS and JavaScript.
- Laravel Dusk: A browser automation and testing tool.
- Laravel Echo: A tool for real-time event broadcasting.
- Laravel Homestead: A virtual machine for Laravel development.
These components enhance the development experience by providing solutions for various aspects of web application creation and deployment.
Modern PHP Development
Laravel has played a pivotal role in modernizing PHP development. It encourages best practices, clean code, and a more structured approach to building web applications. Some of the ways Laravel contributes to modern PHP development include:
-
Composer Integration: Laravel uses Composer, a PHP package manager, to manage project dependencies. This not only simplifies package installation but also ensures that your application uses up-to-date and secure packages.
-
Dependency Injection: Laravel's built-in dependency injection container allows for better organization and testing of code by injecting dependencies as needed, adhering to the SOLID principles.
-
Namespacing: Laravel leverages PHP's namespacing feature, making it easier to organize and manage classes and avoid naming conflicts.
-
Middleware: Middleware allows you to filter HTTP requests entering your application. It's a powerful feature for implementing authentication, security, and other cross-cutting concerns.
-
PSR Standards: Laravel follows the PHP-FIG PSR standards, ensuring compatibility and interoperability with other PHP libraries and frameworks.
-
Modern Routing: Laravel provides a simple and elegant way to define routes and handle HTTP requests. It supports RESTful routing, resource controllers, and more.
Robust Security Measures
Security is a top priority in web development, and Laravel takes it seriously. The framework offers a multitude of built-in security features and follows industry best practices:
-
Authentication and Authorization: Laravel makes implementing user authentication and authorization a breeze. It includes features like user registration, login, password reset, and role-based permissions.
-
CSRF Protection: Cross-Site Request Forgery (CSRF) attacks are thwarted by Laravel's built-in CSRF protection, which automatically generates and validates CSRF tokens.
-
XSS Protection: Laravel escapes user inputs by default, mitigating the risk of Cross-Site Scripting (XSS) attacks.
-
SQL Injection Prevention: Eloquent, Laravel's ORM, uses prepared statements to prevent SQL injection.
-
Rate Limiting and Throttling: Laravel provides tools for rate limiting and request throttling to protect your application from abuse or overuse.
-
Content Security Policy (CSP): Laravel supports CSP headers to prevent unauthorized loading of resources in your application.
-
Security Patches: Laravel releases regular security updates and patches to address vulnerabilities and protect your application.
Now that we've explored the reasons why Laravel is an excellent choice for web development let's dive deeper into the key benefits it offers.
Chapter 3: Key Benefits of Using Laravel
Laravel is known for its rich feature set and developer-friendly tools. In this chapter, we'll discuss ten key benefits of using Laravel and delve into each one in detail.
a. Elegant Syntax and Expressive Code
One of Laravel's most notable advantages is its elegant and expressive syntax. Laravel code is clean and easy to read, making it enjoyable to work with. Here are some aspects of Laravel's syntax that contribute to its elegance:
-
Blade Templating: Laravel's Blade templating engine allows you to write templates that are both concise and expressive. Blade templates use simple and readable syntax, making it easy to create dynamic views.
-
Method Chaining: Laravel uses method chaining extensively, allowing you to chain multiple method calls together, resulting in highly readable and expressive code.
-
Leveraging Modern PHP Features: Laravel embraces the latest features in PHP, including type hinting, namespaces, and closures, which improve code quality and readability.
b. Modular Architecture and Packages
Laravel promotes a modular and organized project structure, enabling developers to build scalable and maintainable applications. Key aspects of this modular architecture include:
-
Service Providers: Service providers bind dependencies, register services, and configure various aspects of your application. They help keep your application's bootstrapping process clean and organized.
-
Packages and Composer: Laravel uses Composer to manage packages and dependencies, making it easy to integrate third-party libraries and extend the functionality of your application.
-
Middleware: Middleware is used for filtering HTTP requests and defining cross-cutting concerns. It allows you to easily add and manage additional functionality, such as authentication and security checks.
c. Built-in Tools and Features
Laravel offers a wide range of built-in tools and features that streamline common development tasks. These include:
-
Task Scheduling: Laravel's built-in task scheduler, powered by the cron, allows you to schedule tasks to run at specified intervals, automating routine tasks.
-
Queues and Workers: Laravel simplifies the handling of time-consuming tasks by providing a robust queue system with support for various queue drivers, including Redis and Amazon SQS.
-
Dependency Injection: Laravel's dependency injection container facilitates the management of class dependencies and promotes the use of interfaces and contracts.
-
Localization and Internationalization: Laravel provides support for translating and formatting content in multiple languages, making your application accessible to a global audience.
d. Blade Templating Engine
Laravel's Blade templating engine is a game-changer when it comes to creating dynamic and reusable views. It offers several benefits, including:
-
Extending Layouts: Blade allows you to create a master layout and extend it in child views, reducing duplication and ensuring a consistent design across your application.
-
Control Structures: Blade supports convenient control structures like loops, conditionals, and includes, making it easy to create dynamic content.
-
Custom Directives: You can define custom Blade directives to encapsulate complex logic into reusable components.
e. Eloquent ORM
Eloquent, Laravel's Object-Relational Mapping (ORM) system, simplifies database interactions. Key advantages of Eloquent include:
-
Active Record Implementation: Eloquent follows the Active Record pattern, where each database table has a corresponding model class, making it easy to work with data.
-
Eloquent Relationships: Eloquent supports relationships like one-to-one, one-to-many, and many-to-many, allowing you to define and query database relationships in a straightforward manner.
-
Database Migrations: Laravel provides a clean and structured way to version control your database schema using migrations.
-
Database Seeding: You can populate your database with sample data using Eloquent's database seeding feature, which is useful for testing and development.
f. Migrations and Database Management
Laravel's database management tools are designed to simplify database-related tasks:
-
Schema Builder: Laravel's schema builder provides a fluent, code-driven way to define your database schema, making it easy to create and modify tables and indexes.
-
Database Seeding: With database seeding, you can populate your database with sample data, making it easier to develop and test your application.
-
Database Transactions: Laravel supports database transactions, allowing you to wrap a series of database operations in a single transaction, ensuring data consistency.
g. Artisan Console
Artisan is a command-line tool that comes with Laravel, offering a wide range of commands for common development tasks:
-
Code Generation: Artisan provides commands to generate boilerplate code for controllers, models, migrations, and more, saving you time and effort.
-
Custom Commands: You can create your own custom Artisan commands to automate repetitive tasks specific to your project.
-
Database Migrations and Seeding: Artisan offers commands for running migrations and seeding databases.
-
Task Scheduling: You can define and schedule tasks using Artisan's task scheduler.
h. RESTful Routing and Controllers
Laravel excels in creating RESTful APIs and web applications due to its robust routing system:
-
RESTful Resource Controllers: Laravel simplifies the creation of RESTful controllers, making it easy to implement CRUD operations for resources.
-
Named Routes: You can assign names to routes, simplifying route generation and URL building.
-
Route Model Binding: Laravel's route model binding automatically injects models into controller methods, reducing the need for manual retrieval.
i. Authentication and Authorization
Laravel streamlines the implementation of user authentication and authorization:
-
Authentication Scaffolding: Laravel provides out-of-the-box authentication scaffolding for login, registration, and password reset, saving you time during development.
-
Customizable Authorization: You can define custom authorization policies and gates to control access to specific actions or resources in your application.
-
User Roles and Permissions: Laravel makes it easy to implement role-based access control (RBAC) with fine-grained permissions.
j. Testing and Debugging
Laravel supports a robust testing ecosystem with tools and features for ensuring your application's reliability:
-
PHPUnit Integration: Laravel integrates PHPUnit for writing and running tests, making it easy to conduct unit, feature, and integration tests.
-
Database Testing: You can use in-memory databases and database transactions for efficient and isolated testing.
-
Test Helpers: Laravel provides a range of helper methods for common testing tasks, such as interacting with the application, asserting responses, and mocking objects.
In the upcoming chapters, we will explore real-world examples of how Laravel's benefits are applied in various applications, demonstrating its versatility and power.
Chapter 4: Real-World Examples of Laravel Applications
a. E-commerce Platforms
E-commerce platforms are some of the most demanding web applications, requiring features like product catalog management, secure payment processing, user accounts, and order tracking. Laravel's features make it an excellent choice for e-commerce projects:
-
Example: Magento Commerce: Magento, a popular e-commerce platform, uses Laravel for its REST API and web services, allowing seamless integration with external systems.
-
Example: Bagisto: Bagisto is an open-source e-commerce platform built on Laravel. It offers a range of features for online store management, including multi-warehouse inventory and dynamic product pricing.
b. Content Management Systems (CMS)
Content management systems are at the heart of content-heavy websites, enabling content creation, publishing, and organization. Laravel's flexibility and user-friendliness make it suitable for building CMS solutions:
-
Example: October CMS: October CMS is a content management system built on Laravel. It provides a simple and elegant platform for creating and managing websites and web applications.
-
Example: Statamic: Statamic is another Laravel-based CMS designed to be user-friendly and developer-friendly. It offers customizable content structures and efficient templating.
c. Social Media Platforms
Social media platforms require real-time interaction, user engagement features, and scalability. Laravel's capabilities make it a solid foundation for creating social networks:
-
Example: PeepSo: PeepSo is a social networking plugin for WordPress that relies on Laravel for scalability and performance enhancements.
-
Example: Edmodo: Edmodo, an education-focused social platform, has utilized Laravel to build its infrastructure, ensuring a robust and secure experience for teachers and students.
d. Enterprise Resource Planning (ERP) Systems
ERP systems manage critical business processes, from inventory and sales to finance and human resources. Laravel's modularity and customizability are advantageous for ERP development:
-
Example: ERPNext: ERPNext is an open-source ERP system that leverages Laravel to provide comprehensive features for small and medium-sized businesses.
-
Example: Odoo: Odoo, a popular open-source ERP and CRM platform, relies on Laravel for a robust and customizable foundation.
e. Educational Portals
Educational portals need to manage courses, content, users, and assessments. Laravel's features and authentication systems suit educational platforms:
-
Example: LearnDash: LearnDash, a leading e-learning platform for WordPress, employs Laravel for its secure and scalable architecture.
-
Example: Open edX: Open edX, an open-source online learning platform used by top universities, utilizes Laravel for performance and security.
f. Project Management Tools
Project management tools require features like task tracking, collaboration, and reporting. Laravel's capabilities make it a great fit for project management software:
-
Example: Taiga: Taiga is an open-source project management platform that uses Laravel to provide a smooth and efficient user experience.
-
Example: Paymo: Paymo, a project management and time-tracking application, relies on Laravel for its web and mobile solutions.
g. Healthcare Information Systems
Healthcare information systems handle patient data, appointments, and clinical records. Laravel's security features and data handling capabilities are invaluable in healthcare applications:
-
Example: Epic Systems: Epic Systems, a major provider of healthcare software, employs Laravel for various components of its systems, ensuring data security and compliance.
-
Example: eClinicalWorks: eClinicalWorks, a leader in healthcare technology, has chosen Laravel for its web-based healthcare solutions.
h. Travel and Booking Applications
Travel and booking applications need to manage reservations, availability, and customer interactions. Laravel's features help build user-friendly and efficient booking platforms:
-
Example: TravelPerk: TravelPerk, a business travel platform, relies on Laravel to power its online booking and travel management services.
-
Example: Agoda: Agoda, a popular travel and accommodation booking platform, employs Laravel for various aspects of its technology stack.
i. Startups and MVPs
Startups often have limited resources and tight deadlines, making rapid development and scalability crucial. Laravel's development speed and flexibility are a perfect fit for startups:
-
Example: StyleSeat: StyleSeat, an online marketplace for beauty and wellness professionals, used Laravel to quickly build and scale its platform.
-
Example: Pabbly: Pabbly, a subscription management platform, leveraged Laravel for its Minimum Viable Product (MVP) to get to market faster.
j. Ecosystem and Community Projects
Laravel's popularity has led to a wide range of community projects, open-source tools, and extensions. These community-driven initiatives showcase the adaptability and versatility of Laravel:
-
Example: Laravel Horizon: Laravel Horizon is a queue management and monitoring tool for Laravel applications.
-
Example: Laravel Telescope: Laravel Telescope is an elegant and powerful debug assistant for Laravel applications.
These real-world examples demonstrate the diverse applications of Laravel across different industries and use cases. Whether you're building an e-commerce platform, an ERP system, or a social media network, Laravel's features and capabilities make it a versatile choice for web development.
In the next chapter, we will explore performance optimization techniques in Laravel, helping you ensure that your application is both fast and responsive.
Chapter 5: Performance Optimization in Laravel
Performance optimization is a critical aspect of web development, ensuring that your application runs smoothly, loads quickly, and can handle a high volume of traffic. Laravel offers several features and techniques for optimizing the performance of your web applications.
Caching and Performance Enhancement
Caching is a technique for storing frequently used data in memory or a faster storage system to reduce the time it takes to retrieve that data. Laravel provides multiple caching mechanisms, including:
-
Database Query Caching: Laravel allows you to cache the results of database queries, reducing the load on your database and speeding up responses.
-
Page Caching: You can cache entire HTML pages, bypassing the PHP and Laravel execution process for static content.
-
Opcode Caching: PHP opcode caching, such as OPcache, can significantly boost the performance of PHP applications, including Laravel.
Database Query Optimization
Database queries are often a bottleneck in web applications. Laravel provides tools and techniques to optimize database queries:
-
Eloquent Performance Tips: Use Eloquent's lazy loading, select only the necessary columns, and eager load relationships to reduce the number of database queries.
-
Query Indexing: Proper indexing of database tables can dramatically improve query performance. Laravel's migration system makes it easy to define indexes.
-
Database Sharding and Replication: For high-traffic applications, consider database sharding to distribute the load across multiple databases and replication to improve read performance.
-
Use Caching: Cache frequently retrieved data to reduce the number of database queries.
Scalability and Load Balancing
As your application grows, you may need to scale it horizontally by adding more servers. Laravel supports horizontal scalability and load balancing:
-
Load Balancers: Load balancers distribute incoming traffic across multiple web servers, ensuring optimal resource utilization.
-
Queue Workers: Use Laravel's queue system to process time-consuming tasks asynchronously, improving the responsiveness of your application.
-
Content Delivery Networks (CDNs): CDNs can distribute static assets like images and stylesheets, reducing the load on your web server.
-
Database Clustering: Implement database clustering solutions to distribute the database load across multiple servers.
Optimizing performance is an ongoing process. By implementing caching, optimizing database queries, and planning for scalability, you can ensure that your Laravel application remains responsive and efficient, even as it grows.
In the next chapter, we'll delve into security features and best practices in Laravel, helping you protect your application and user data from threats.
Chapter 6: Security Features and Best Practices
Security is paramount in web development, and Laravel offers a wide range of features and best practices to help protect your application from common threats and vulnerabilities.
a. Authentication and Authorization
Laravel simplifies user authentication and authorization with features such as:
-
Authentication Scaffolding: Laravel's built-in authentication scaffolding provides pre-built registration, login, and password reset functionality, saving you time and effort.
-
Middleware for Authorization: Use middleware to control access to routes and actions based on user roles and permissions.
-
Custom Policies and Gates: Define custom authorization policies and gates for fine-grained control over access to specific resources and actions.
b. Input Validation
Input validation is a crucial aspect of security. Laravel provides tools to validate user input and prevent common security vulnerabilities:
-
Validation Rules: Use Laravel's validation rules to check input data for correctness, preventing issues like SQL injection and cross-site scripting.
-
Cross-Site Request Forgery (CSRF) Protection: Laravel automatically generates and validates CSRF tokens to protect against cross-site request forgery attacks.
c. Cross-Site Request Forgery (CSRF) Protection
CSRF attacks occur when an attacker tricks a user into making an unwanted action on your application. Laravel's CSRF protection helps mitigate this threat:
-
Automatic CSRF Tokens: Laravel generates and verifies CSRF tokens for each form submitted, preventing unauthorized actions.
-
X-CSRF-Token Header: Protect your application's API by including the X-CSRF-Token header in AJAX requests.
d. Cross-Site Scripting (XSS) Prevention
Cross-Site Scripting (XSS) attacks can inject malicious scripts into web pages. Laravel helps prevent XSS attacks with:
-
Automatic Data Escaping: Laravel escapes user inputs by default, making it difficult for attackers to inject malicious scripts.
-
Blade Directives: Use Blade directives like
@{{ $variable }}
for output that should not be escaped, and@{{!! $variable !!}}
for raw HTML output.
e. SQL Injection Prevention
SQL injection is a severe security vulnerability that allows attackers to execute arbitrary SQL queries. Laravel's Eloquent ORM and query builder protect against SQL injection:
-
Prepared Statements: Eloquent and the query builder use prepared statements to bind parameters securely and prevent SQL injection.
-
Query Parameterization: Avoid direct SQL query construction and use parameterized queries to mitigate SQL injection risks.
f. Rate Limiting and Throttling
Laravel includes rate limiting and throttling features to prevent abuse and protect your resources:
-
Middleware for Rate Limiting: Apply rate limiting to API routes and control the number of requests a user can make in a given time frame.
-
Throttle API Requests: Protect your API by throttling requests based on client IP address or authenticated user.
g. Security Updates and Patches
Laravel maintains a strong commitment to security. It regularly releases security updates and patches to address vulnerabilities and protect your application. Keeping your Laravel installation up-to-date is crucial for maintaining a secure web application.
By implementing these security features and best practices, you can significantly enhance the security of your Laravel application, safeguarding your data and users from common web threats.
In the next chapter, we'll explore how Laravel can be seamlessly integrated with other technologies, allowing you to create more powerful and versatile applications.
Chapter 7: Integrating Laravel with Other Technologies
Laravel's flexibility and adaptability make it an ideal choice for integrating with a wide range of other technologies. In this chapter, we'll explore various integration scenarios that allow you to extend the capabilities of your Laravel application.
a. Laravel and JavaScript Frameworks (Vue.js, React)
Modern web development often involves integrating client-side JavaScript frameworks with the server-side framework. Laravel provides excellent support for this integration:
-
Vue.js and Laravel: Laravel offers first-class support for Vue.js, allowing you to build reactive and dynamic user interfaces using Vue components.
-
React and Laravel: You can seamlessly integrate React into your Laravel application to create interactive and responsive web interfaces.
b. RESTful APIs and Microservices
Laravel is well-suited for building and consuming RESTful APIs and microservices:
-
Building RESTful APIs: Laravel's routing and controller capabilities make it easy to create RESTful APIs for your application, allowing external services to interact with your system.
-
Consuming RESTful APIs: Laravel's HTTP client provides an elegant and expressive way to interact with external APIs, whether you're retrieving data or sending requests.
c. Docker and Containerization
Docker and containerization have revolutionized the way applications are deployed and managed. Laravel can be easily containerized:
-
Docker and Laravel: You can create Docker containers for your Laravel application, simplifying deployment and ensuring consistency across environments.
-
Kubernetes Integration: By containerizing your Laravel application, you can leverage Kubernetes for container orchestration, scaling, and management.
d. Cloud Services (AWS, Azure, GCP)
Laravel applications can be deployed on popular cloud platforms like AWS, Azure, and Google Cloud Platform (GCP):
-
Laravel on AWS: Deploy your Laravel application on Amazon Web Services (AWS) to take advantage of scalable infrastructure and services.
-
Laravel on Azure: Microsoft Azure provides a robust platform for hosting Laravel applications, with services like Azure App Service and Azure SQL Database.
-
Laravel on GCP: Google Cloud Platform offers a range of services for deploying and managing Laravel applications, including Google App Engine and Cloud SQL.
e. CI/CD Pipelines
Continuous Integration and Continuous Deployment (CI/CD) pipelines automate the testing and deployment of your Laravel applications:
-
CI/CD Tools: Popular CI/CD tools like Jenkins, Travis CI, and GitLab CI/CD can be configured to build, test, and deploy Laravel applications automatically.
-
Docker and CI/CD: Combining Docker with CI/CD pipelines simplifies the process of deploying Laravel applications to various environments.
By integrating Laravel with other technologies, you can enhance your application's functionality, scalability, and deployment process, ultimately creating a more versatile and powerful solution.
In the next chapter, we'll explore how to extend Laravel's capabilities by incorporating Composer packages, allowing you to easily integrate third-party functionality into your application.
Chapter 8: Extending Laravel with Composer Packages
Laravel's extensibility is one of its strongest features, thanks to its integration with Composer, a PHP package manager. In this chapter, we'll explore the world of Composer packages and how they can enhance your Laravel application.
a. Awe-Inspiring Laravel Packages
Laravel's rich ecosystem includes numerous packages that cater to a wide range of functionalities. Here are some notable Laravel packages and their use cases:
-
Laravel Passport: Laravel Passport provides a simple and efficient way to implement OAuth2 authentication in your applications, making it ideal for building APIs.
-
Laravel Scout: Laravel Scout is an elegant solution for adding full-text search to your Laravel applications, using powerful search engines like Algolia.
-
Laravel Cashier: Laravel Cashier simplifies subscription billing and management in your application, perfect for SaaS platforms.
-
Laravel Telescope: Laravel Telescope is a must-have developer tool for debugging and monitoring your application, offering insights into requests, exceptions, and more.
-
Laravel Horizon: Laravel Horizon is a powerful queue dashboard and monitoring tool that enhances your application's job processing capabilities.
b. Installation and Usage
Integrating Composer packages into your Laravel application is straightforward:
-
Require a Package: Use Composer to require a package by specifying its name and version in your
composer.json
file. -
Update Dependencies: Run
composer update
to fetch and install the required package. -
Service Providers: Most Laravel packages come with service providers that you need to register in the
config/app.php
configuration file. Service providers help your application discover and use the package's features. -
Configuration: Some packages may require you to publish configuration files for customization. You can do this using the
vendor:publish
Artisan command. -
Usage: Follow the package's documentation to use its features within your Laravel application.
Composer packages not only enhance your application's functionality but also save you time by leveraging pre-built solutions for common tasks. They are a valuable asset in Laravel development, allowing you to focus on building your application's unique features.
In the next chapter, we'll explore how Laravel can be applied in Internet of Things (IoT) and real-time applications, expanding its use cases to the forefront of technology.
Chapter 9: Laravel's Role in IoT and Real-Time Applications
Laravel's versatility extends beyond traditional web applications, making it a suitable choice for emerging technologies like the Internet of Things (IoT) and real-time applications. In this chapter, we'll explore how Laravel can be leveraged in these domains.
a. WebSockets and Broadcasting
Laravel's integration with WebSockets allows real-time communication between the server and clients. Key components include:
-
Laravel Echo: Laravel Echo is a JavaScript library that simplifies subscribing to and broadcasting events in real time.
-
Laravel Broadcasting: Broadcasting is the process of broadcasting events to connected clients using WebSocket and Redis, making it ideal for real-time applications.
-
Presence Channels: Laravel's presence channels enable you to track the presence of users within a channel, facilitating chat applications and collaborative tools.
b. IoT Data Collection and Management
Laravel can play a pivotal role in managing and processing data from IoT devices:
-
Data Ingestion: Laravel can ingest data from IoT sensors and devices through APIs, MQTT, or other communication protocols.
-
Data Storage: Laravel's database features, combined with NoSQL databases, can efficiently store and manage large volumes of IoT data.
-
Data Processing: Use Laravel's background job processing to perform data analytics and derive valuable insights from IoT data.
-
Real-Time Monitoring: Leverage real-time features to provide monitoring and alerts for IoT devices and systems.
c. Real-Time Notifications
Real-time notifications are essential in various applications, including social media, collaborative tools, and IoT:
-
Laravel Notifications: Laravel provides a notification system that can be integrated with real-time broadcasting, enabling instant alerts and updates.
-
Push Notifications: Laravel can be integrated with services like Firebase Cloud Messaging (FCM) to send push notifications to mobile devices.
-
Alerting and Monitoring: Laravel's real-time capabilities can be used to build monitoring and alerting systems for various applications, including IoT.
The ability to handle real-time data and events opens up new opportunities for Laravel in applications that require instant communication, data processing, and alerts.
In the next chapter, we'll explore how you can continue learning and receive support from the Laravel community to excel in Laravel development.
Chapter 10: Continuous Learning and Community Support
Laravel's success can be attributed not only to its features and capabilities but also to its active community and commitment to education. In this chapter, we'll look at the resources available to continue learning and get support for your Laravel projects.
a. Laravel Documentation
The Laravel documentation is a treasure trove of information and guidance for developers. It covers everything from installation and configuration to in-depth explanations of Laravel's features and components. The documentation is continually updated and is an essential resource for developers of all skill levels.
b. Online Courses and Tutorials
Numerous online courses and tutorials are available to help you master Laravel. These courses cover a wide range of topics, from the basics of Laravel development to advanced techniques and best practices. Platforms like Laravel University, Laracasts, Udemy, and YouTube offer a plethora of courses to choose from.
c. Laravel Conferences and Events
The Laravel community is vibrant and actively participates in conferences and events. Attending Laravel-specific events can provide you with the opportunity to learn from experts, network with other developers, and stay up-to-date with the latest trends and technologies in the Laravel ecosystem.
d. Active Forums and Community Resources
The Laravel community is active on forums, discussion boards, and social media platforms. Websites like the Laravel.io forum and the Laravel subreddit are excellent places to ask questions, seek advice, and share your knowledge. The community is welcoming and always ready to help fellow developers.
e. Contributions and Open-Source Projects
Contributing to open-source Laravel projects is an excellent way to hone your skills and give back to the community. Laravel's GitHub repository and other related repositories offer opportunities to contribute code, documentation, or bug reports.
f. Package Development
Building and sharing your own Composer packages for Laravel is a valuable way to contribute to the community and demonstrate your expertise. Many popular Laravel packages were created by community members.
g. Laravel Certification
The Laravel Certification program provides official recognition of your Laravel skills. Becoming a Laravel Certified Developer is a great way to showcase your expertise and boost your career.
By taking advantage of these resources and actively participating in the Laravel community, you can continue to grow and excel in Laravel development. Laravel's community is known for its support and knowledge sharing, making it a great environment for both beginners and experienced developers.
Chapter 11: Case Studies: Success Stories with Laravel
In this chapter, we'll delve into case studies to highlight the success stories of companies and developers who have embraced Laravel for their projects. These case studies showcase the practical application of Laravel in real-world scenarios.
a. Taylor Otwell's Journey
Taylor Otwell, the creator of Laravel, is a prominent figure in the Laravel community. His journey from developing a simple PHP project to creating one of the most popular PHP frameworks in the world is an inspiring story of passion and perseverance.
Taylor's dedication to Laravel and its ongoing development has played a crucial role in the framework's success.
b. Laravel-Powered Startups
Laravel has been the foundation for numerous successful startups, allowing them to rapidly develop and deploy their Minimum Viable Products (MVPs). Startups like StyleSeat, Pabbly, and others have leveraged Laravel's speed and flexibility to enter the market quickly and efficiently.
c. Enterprise Success Stories
Large enterprises have also adopted Laravel for their critical applications. Companies like Magento Commerce, Epic Systems, and others rely on Laravel's capabilities to meet the demands of their complex and high-traffic projects.
d. Community Projects and Initiatives
The Laravel community has initiated and maintained various open-source projects and packages that have gained popularity and are widely used by developers worldwide. Projects like Laravel Horizon, Laravel Telescope, and others demonstrate the community's commitment to enhancing Laravel's ecosystem.
These case studies emphasize the adaptability and versatility of Laravel across different scales and industries, from individual developers and startups to large enterprises and community-driven initiatives.
Chapter 12: Laravel: A Framework for the Future
In this final chapter, we'll reflect on Laravel's position in the web development landscape and its potential for the future. Laravel's growth, continuous improvement, and adaptability make it a framework that's poised to remain a significant player in the industry.
a. Laravel's Growth and Popularity
Laravel's growth trajectory has been remarkable. It has risen from a new entrant in the PHP framework space to one of the most popular and widely used frameworks in the world. Its active community and continuous development ensure that Laravel stays up-to-date with industry trends and best practices.
b. The Future of PHP and Laravel
PHP continues to evolve with each new version, introducing performance improvements and modern features. Laravel aligns itself with the latest PHP developments, ensuring that developers have access to the most advanced capabilities in their projects.
c. Expanding Use Cases
As we've seen in the case studies, Laravel is not limited to any specific industry or application. Its versatility allows it to be applied in a wide range of scenarios, from e-commerce and content management to real-time applications and IoT projects.
d. Laravel as a Catalyst for Developer Success
Laravel has played a significant role in the success of developers, whether they are starting their careers, building startups, or working on enterprise projects. Its developer-friendly features, comprehensive documentation, and supportive community make it an ideal framework for developers to achieve their goals.
e. The Laravel Ecosystem
Laravel's ecosystem continues to grow with the introduction of new packages, tools, and integrations. These additions enhance Laravel's capabilities and offer developers a broader set of solutions for their projects.
Laravel's journey is a testament to its commitment to innovation, adaptability, and community-driven development. As it continues to evolve, Laravel remains a framework for the future, equipping developers with the tools they need to build exceptional web applications.
Conclusion
In this extensive guide, we've explored the Laravel framework, its features, benefits, and real-world applications. We've delved into the reasons why Laravel is a top choice for web development, from its elegant syntax and robust security to its modularity and real-time capabilities.
Laravel's success is not only due to its features but also its active community, continuous learning resources, and a thriving ecosystem of packages and integrations. Its adaptability across various industries and use cases, from e-commerce to IoT, showcases its versatility and power.
Whether you're a developer just starting your journey or an experienced professional looking for the right framework for your next project, Laravel is a framework that empowers you to build exceptional web applications.
As Laravel continues to grow and adapt to emerging technologies, it remains a framework for the future, supporting the success of developers and the development of innovative web solutions.
What's Your Reaction?
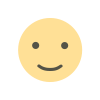
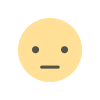
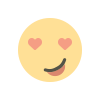
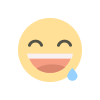
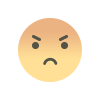
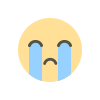
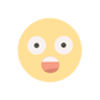